Creating Tokens on the Ecrox Blockchain : involves several steps, including writing a smart contract, deploying it to the blockchain, and verifying its functionality.
Below is a step-by-step guide on how to create token on ecrox20:
Prerequisites:
Ecrox Wallet: Make sure you have an Ecrox wallet with some Ecrox tokens to pay for transaction fees.
Development Environment: Install Node.js, npm, and any other necessary development tools.
Ecrox Blockchain Network Access: Ensure you have access to the Ecrox blockchain network, either via a local node or an online provider.
Steps to Create an ECROX20 Token
Write the ECROX20 Smart Contract
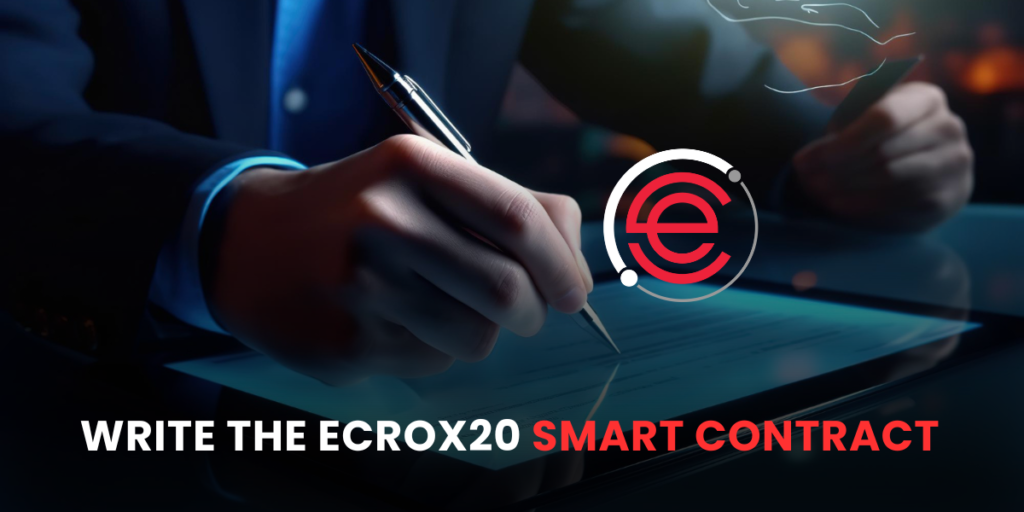
Create a new file for your token smart contract, for example, Ecrox20Token.sol, and write the following code:
solidity
Copy code
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract Ecrox20Token {
string public name = “YourTokenName”;
string public symbol = “YTN”;
uint8 public decimals = 18;
uint256 public totalSupply;
mapping(address => uint256) public balanceOf;
mapping(address => mapping(address => uint256)) public allowance;
event Transfer(address indexed from, address indexed to, uint256 value);
event Approval(address indexed owner, address indexed spender, uint256 value);
constructor(uint256 _initialSupply) {
totalSupply = _initialSupply * (10 ** uint256(decimals));
balanceOf[msg.sender] = totalSupply;
}
function transfer(address _to, uint256 _value) public returns (bool success) {
require(balanceOf[msg.sender] >= _value, "Insufficient balance");
balanceOf[msg.sender] -= _value;
balanceOf[_to] += _value;
emit Transfer(msg.sender, _to, _value);
return true;
}
function approve(address _spender, uint256 _value) public returns (bool success) {
allowance[msg.sender][_spender] = _value;
emit Approval(msg.sender, _spender, _value);
return true;
}
function transferFrom(address _from, address _to, uint256 _value) public returns (bool success) {
require(_value <= balanceOf[_from], "Insufficient balance");
require(_value <= allowance[_from][msg.sender], "Allowance exceeded");
balanceOf[_from] -= _value;
balanceOf[_to] += _value;
allowance[_from][msg.sender] -= _value;
emit Transfer(_from, _to, _value);
return true;
}
}
Compile the Smart Contract
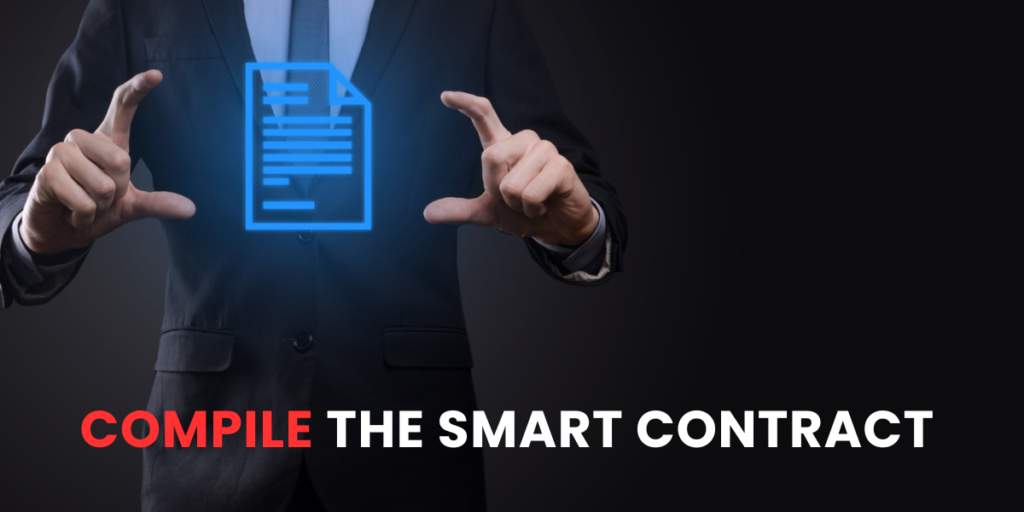
Use the Solidity compiler (solc) to compile your smart contract. You can use tools like Remix, Truffle, or Hardhat for this purpose.
Deploy the Smart Contract
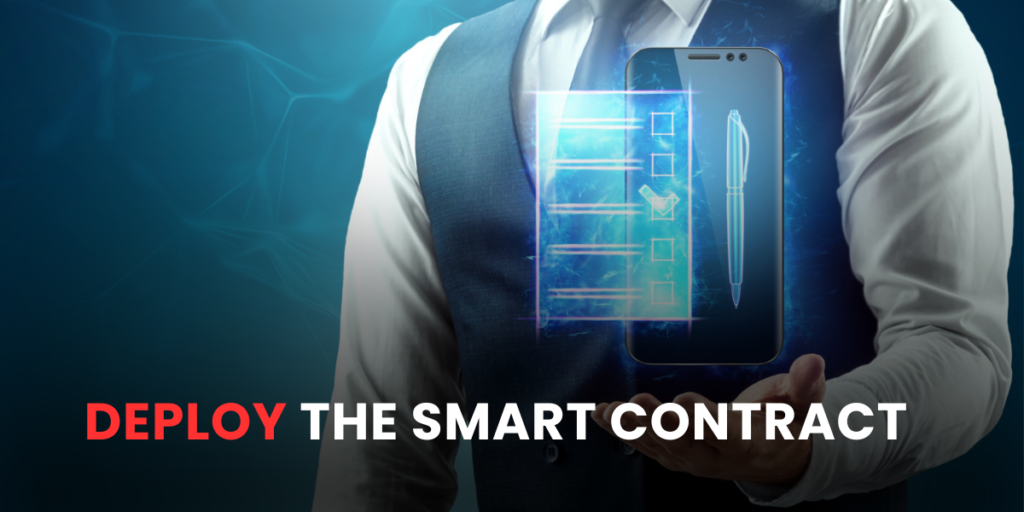
You need to deploy the compiled smart contract to the Ecrox blockchain. Here’s an example using Web3.js and Truffle:
Install Web3.js and Truffle:
bash
Copy code
npm install web3 truffle
Create Deployment Script:
Create a deployment script, for example, deploy.js:
javascript
Copy code
const Web3 = require(‘web3’);
const fs = require(‘fs’);
const path = require(‘path’);
// Connect to Ecrox blockchain (replace with your provider URL)
const providerUrl = ‘https://your-ecrox-provider-url’;
const web3 = new Web3(new Web3.providers.HttpProvider(providerUrl));
// Read the compiled contract
const contractPath = path.resolve(__dirname, ‘build/contracts/Ecrox20Token.json’);
const contractJson = JSON.parse(fs.readFileSync(contractPath, ‘utf8’));
// Get contract ABI and bytecode
const { abi, bytecode } = contractJson;
const deployContract = async () => {
const accounts = await web3.eth.getAccounts();
const deployerAccount = accounts[0];
const ecrox20Token = new web3.eth.Contract(abi);
ecrox20Token.deploy({
data: bytecode,
arguments: [1000000] // Initial supply
})
.send({
from: deployerAccount,
gas: 1500000,
gasPrice: '30000000000'
})
.on('receipt', (receipt) => {
console.log('Contract deployed at address:', receipt.contractAddress);
})
.on('error', (error) => {
console.error('Deployment error:', error);
});
};
deployContract();
Run Deployment Script:
bash
Copy code
node deploy.js
Verify the Token
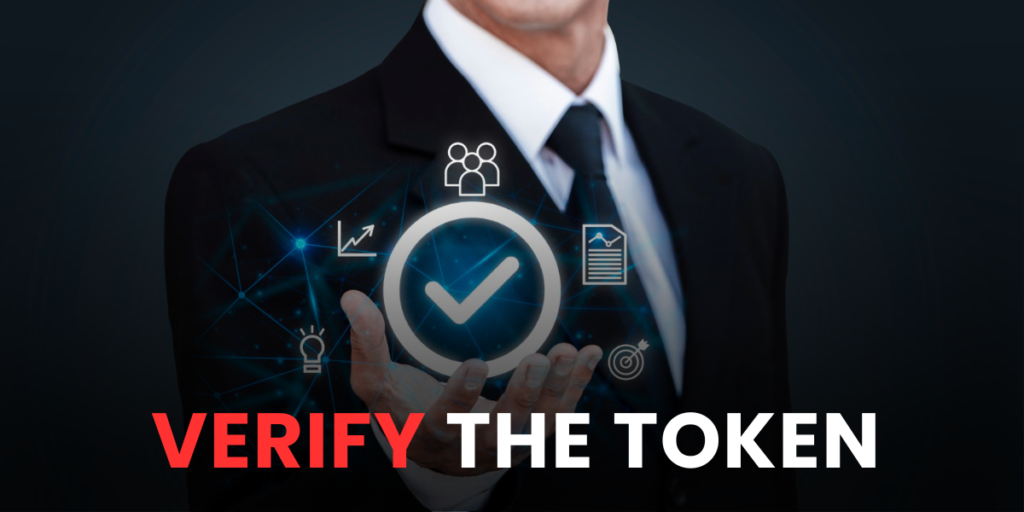
After deploying the contract, verify that it is functioning correctly by interacting with it through Web3.js, Truffle, or Remix. You can perform actions such as checking balances, transferring tokens, and approving allowances.
Additional Considerations
Security: Ensure your smart contract is audited and follows best practices to prevent vulnerabilities.
Regulation: Be aware of and comply with any regulatory requirements for issuing tokens.
Testing: Thoroughly test your contract on a test network before deploying to the mainnet.
By following these steps, you should be able to create and deploy an ECROX20 token on the Ecrox blockchain successfully.
Conclusion:
Creating Tokens on the Ecrox20 Blockchain involves several critical steps, including writing the smart contract, compiling it, deploying it to the blockchain, and verifying its functionality. By following the outlined guide and ensuring adherence to best practices for security and testing, you can successfully launch your token. Always remain aware of regulatory requirements to maintain compliance.
FAQs:
What is an ECROX20 token?
An ECROX20 token is a standard token on the Ecrox blockchain, similar to the ERC20 standard on Ethereum. It defines a set of rules for token interactions, ensuring compatibility and interoperability across the Ecrox ecosystem.
What tools do I need to create an ECROX20 token?
You’ll need an Ecrox wallet, a development environment with Node.js and npm, and access to the Ecrox blockchain network. Additionally, you’ll use tools like Solidity, Web3.js, and Truffle or Hardhat for contract development and deployment.
How do I compile my ECROX20 smart contract?
You can use tools like Remix, Truffle, or Hardhat to compile your Solidity smart contract. These tools will convert your Solidity code into bytecode that can be deployed to the blockchain.
How do I deploy my ECROX20 smart contract?
After compiling your contract, use Web3.js and Truffle (or similar tools) to deploy it to the Ecrox blockchain. You’ll need to connect to an Ecrox provider, get your contract’s ABI and bytecode, and execute the deployment script.
How can I verify my token’s functionality?
Verify your token by interacting with it using Web3.js, Truffle, or Remix. Check balances, perform transfers, and approve allowances to ensure the contract operates as expected.
What security measures should I consider when creating a token?
Ensure your smart contract follows best practices to prevent vulnerabilities. Consider getting your contract audited by professionals, and thoroughly test it on a test network before deploying to the mainnet.
Are there any regulatory requirements for issuing tokens?
Yes, depending on your jurisdiction, there may be regulatory requirements for issuing tokens. It’s crucial to be aware of these requirements and ensure compliance to avoid legal issues.